In This Topic
Connected characters and oversampled characters
In This Topic
Sometimes characters in documents appear thick and their features unclear, like if there was too much ink while printing them. This is usually the result of repeated scanning and printing of the document, especially using the low setting such as DPI. Erosion can help fix this issue.
Before / After
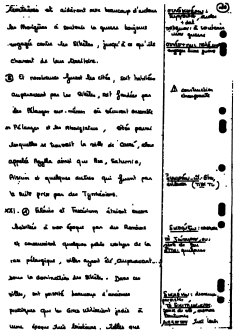
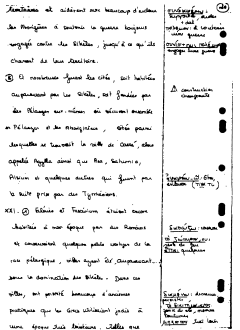
Here is the code example how it works.